Examples from the GitHub API
Now that we know about requests, documents, queries, and fields, let’s put this knowledge to use and explore some real-world examples of GraphQL requests that you can ask the GitHub API for. GitHub moved from REST APIs to GraphQL APIs in 2017. You can explore the new GitHub API at https://developer.github.com. You need to be logged in (with a GitHub.com account) to use this API and it is subject to rate limiting.
This GitHub API makes use of your real, live, production data at GitHub.com |
Under the "API Docs" menu, you should see both the GraphQL API and the REST API. Navigate to the GraphQL API and then to the Explorer tab. Once you are logged into github.com, you should see an embedded GraphiQL editor that will enable you to explore the GitHub GraphQL API.
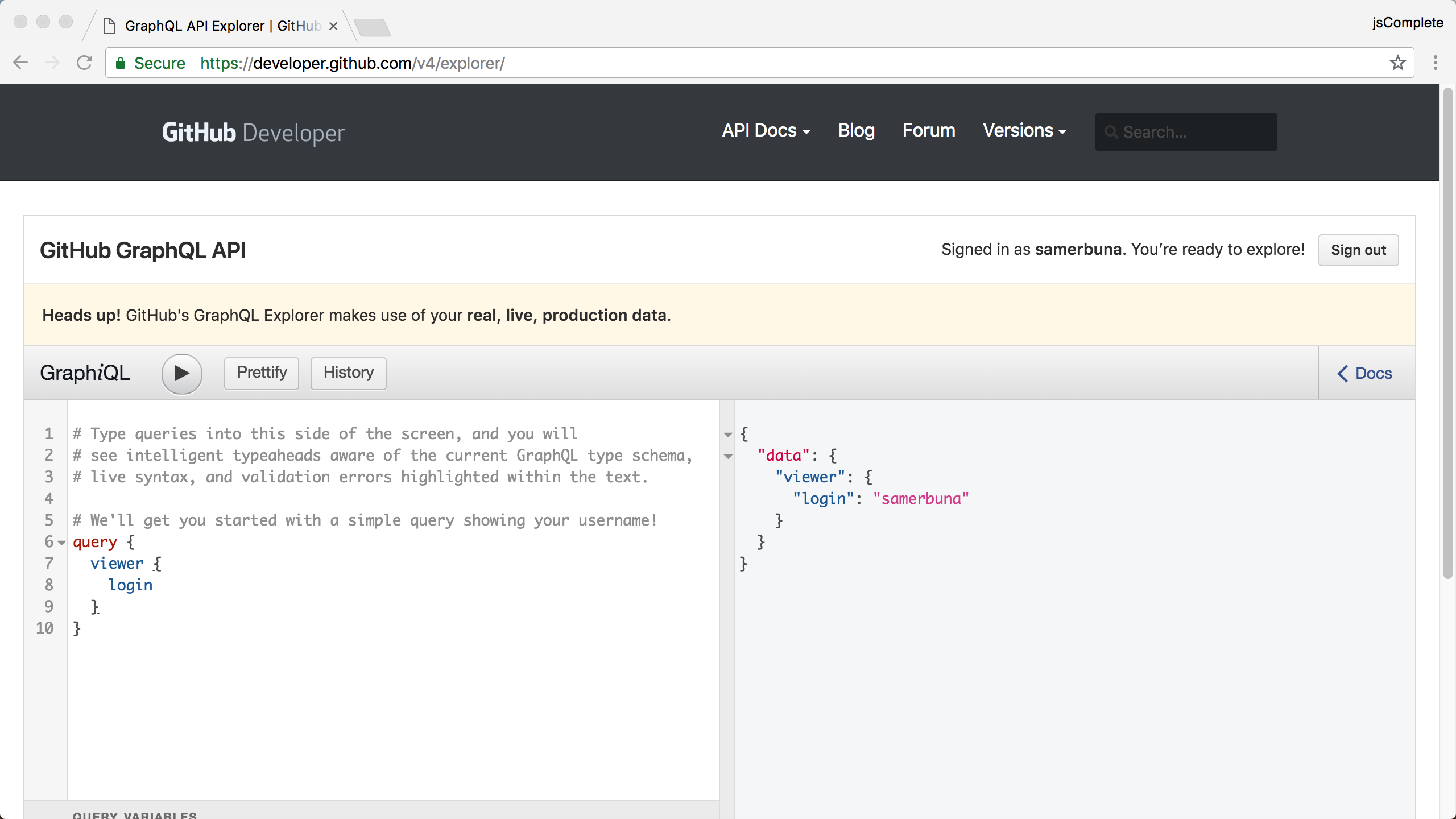
Let’s first look at some common queries from this API.
1. Reading Data From GitHub
When you first launch the GitHub GraphQL API explorer, it has a default simple query that will display your own login. The currently logged-in user is represented by the field “viewer”. Under this field, you can read all the information that is available about you at GitHub.
For example, here is a query to see information about the last 10 repositories that you own or contribute to:
{ viewer { repositories(last: 10) { nodes { name description } } } }
Here is another query to see all the supported licenses in GitHub along with their URLs:
{ licenses { name url } }
Here is a more complex query to find the first 10 issues of the "facebook/graphql" repository. It asks for the name of the author and the title that was used for the issue page along with the date that issue was created:
{ repository(owner: "facebook", name: "graphql") { issues(first: 10) { nodes { title createdAt author { login } } } } }
2. Updating Data at GitHub
Let’s now explore some mutations we can do with the GitHub GraphQL API. The simplest mutation is to "star" a repo. Here is a mutation which, if you execute under your logged-in user, its action would be equivalent to you going to github.com/facebook/graphql and clicking the "star" button:
mutation { addStar(input: {starrableId: "MDEwOlJlcG9zaXRvcnkzODM0MjIyMQ=="}) { (1) starrable { stargazers { totalCount } } } }
1 | Use listing 7.6 to find this starrableId value |
The mutation will star the repo and then read the new total number of stargazers after the mutation. The input for this mutation is a simple object that has a starrableId
value, which is the node identifier for the "graphql" repository. I was able to find that value using this query:
{ repository(name: "graphql", owner: "facebook") { id } }
Let’s execute another mutation. This time let’s add a comment to an issue in a repository. I created an issue for you to test this mutation under the repository at github.com/jscomplete/graphql-in-action.
You can see the details of this issue that I am about to comment on for the first time using this query:
query GetIssueInfo { repository(owner: "jscomplete", name: "graphql-in-action") { issue(number: 1) { id title } } }
This will give you the value of the id
field that is needed to add a comment to the issue using a mutation. Now you need to execute the following mutation that uses the id
value you found using the query in listing 7.5.
mutation AddCommentToIssue { addComment(input: { subjectId: "MDU6SXNzdWUzMDYyMDMwNzk=", body: "Hello GraphQL" }) { commentEdge { node { createdAt } } } }
After the mutation in listing 7.7 saves your comment to the special issue, it will report the createdAt
date for that comment. Feel free to send as many comments as you wish to this special issue, but only do so through the GraphQL API explorer 😊.
You can see the comments you added and all the other comments on this issue at https://github.com/jscomplete/graphql-in-action/issues/1.
3. Introspective Queries
GraphQL APIs support introspective queries that can be used to answer questions about the API schema itself. This introspection support enables GraphQL tools to have powerful features and it is what drives the features we have been using in the GraphiQL editor. For example, the awesome type-ahead list in GraphiQL is sourced with an introspective query.
Introspective queries start with a top-level field that’s either __type
or __schema
, which are known as meta-fields. There is also another meta-field named __typename
that can be used to retrieve the name of any object type. Fields with names that begin with double underscore characters are reserved for introspection support.
Meta-fields are implicit, which means they do not appear in the fields list of their types. |
The __schema
field can be used to read information about the API schema, like what types and directives it supports. We will explore directives soon.
Let’s ask the GraphQL API schema what types it supports. Here is an introspective query for that:
{ __schema { types { name description } } }
This query will return all the types this schema supports and it will also include each type’s description. This is a helpful list to explore the custom types defined in the GitHub GraphQL schema. For example, you should see that this schema defines types like Repository
, Commit
, Project
, Issue
, PullRequest
, and many more.
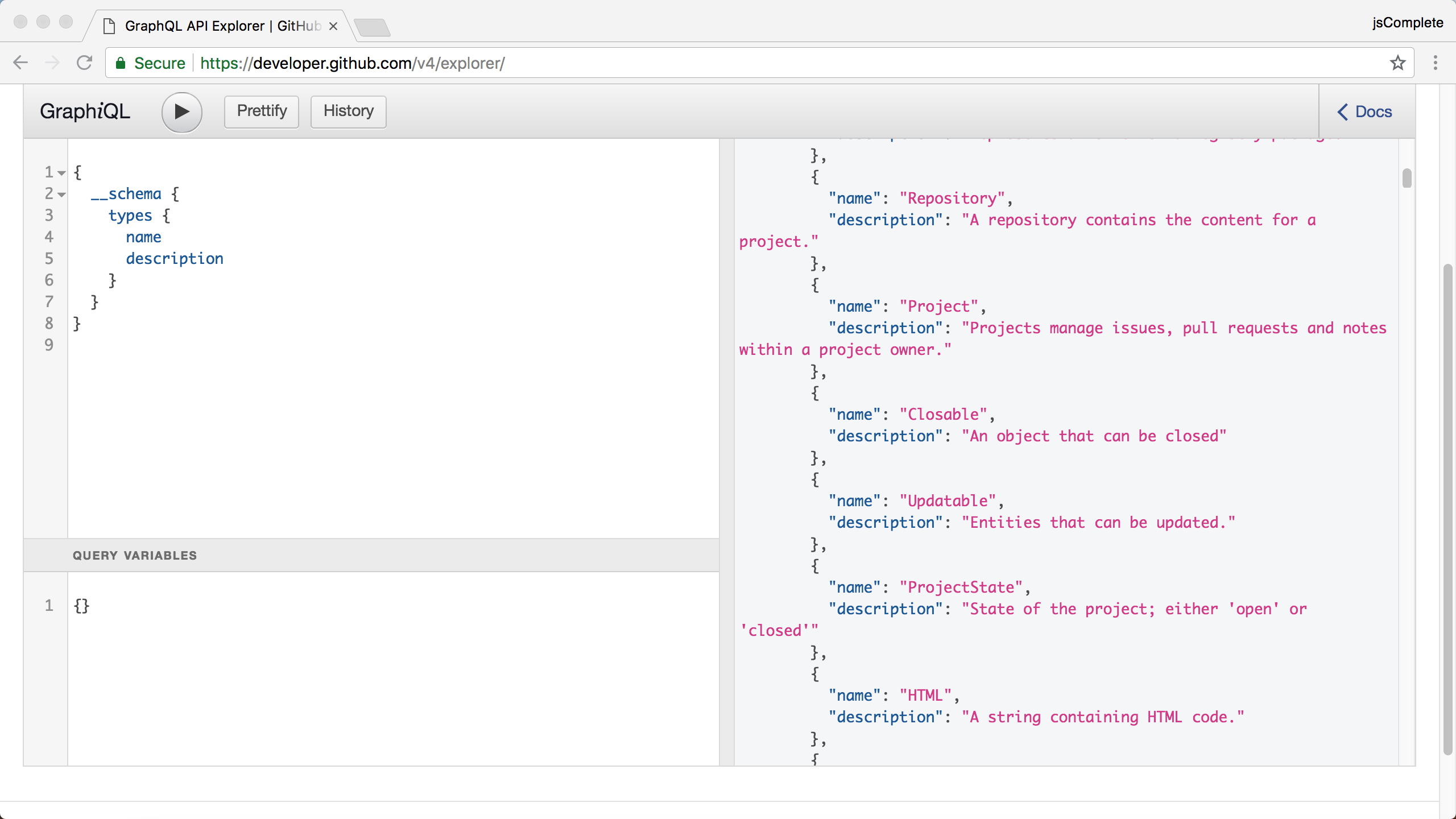
If you need to retrieve information about a single type, you can use the __type
meta-field. For example, here is a query to find all the supported fields under the type “Commit” along with any arguments they accept:
{ __type(name: "Commit") { fields { name args { name } } } }
Use the GraphiQL type-ahead feature to discover what other information you can ask for under these introspective meta-fields.