Object Literals
You can create a JavaScript object in a few different ways but the most common way is to use an object literal:
const obj = { // key: value };
This is easier than doing something like new Object()
, which you can do if you want to.
Literal notation (AKA initializer notation) is very common. We use it for objects, arrays, strings, numbers, and even things like regular expressions:
const obj = {}; // Object() const arr = []; // Array() const str = ""; // String() const num = 0; // Number() const reg = /(?:)/; // RegExp()
Here are a few things you can with object literals:
const mystery = 'answer'; const InverseOfPI = 1 / Math.PI; const obj = { p1: 10, // Plain old object property (don't abbreviate) f1() {}, // Define a shorthand function property InverseOfPI, // Define a shorthand regular property f2: () => {}, // Define an arrow function property [mystery]: 42, // Define a dynamic property };
Did you notice that [mystery]
thing? That is NOT an array or a destructuring thing (destructuring is explained next). It is how you define a dynamic property.
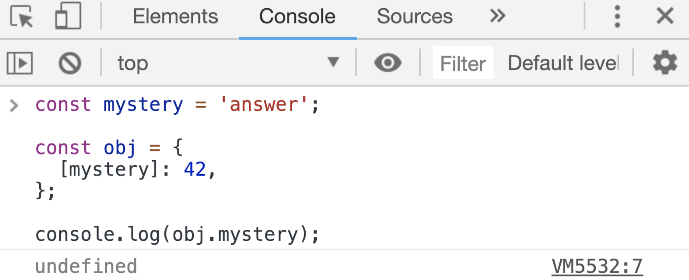
When you use the dynamic property syntax, JavaScript will first evaluate the expression inside []
and whatever that expression evaluates to becomes the object’s property.
For the example above, the obj
object will have a property “answer
” with the value of 42
.
Another widely popular feature about object literals is available to you when you need to define an object with property names to hold values that exist in the current scope with the exact same names. You can use the shorthand property name syntax for that.
That’s what we did for the InverseOfPI
variable above. That part of the object is equivalent to:
const obj = { InverseOfPI: InverseOfPI, };
Objects are very popular in JavaScript. They are used to manage and communicate data and using these features will make the code a bit shorter and easier to read.