Destructuring Arrays and Objects
The destructuring syntax is simple but it makes use of the same curly and square brackets you use with object/array literals, which makes it confusing sometimes. You need to inspect the context to know whether a set of curly brackets ({}
) or square brackets ([]
) are used as literal initializing or destructuring assignment.
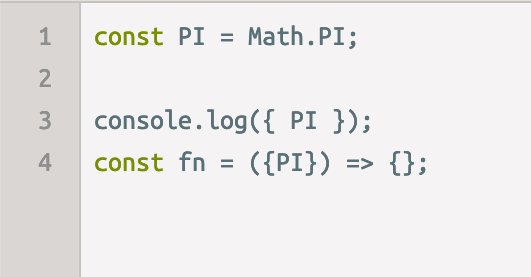
In the code above, the { PI }
on line 3 is an object literal while the {PI}
on line 4 is used as a destructuring assignment.
It can really get a lot more complicated than that, but here are 2 simple general rules to identify what’s what:
-
If brackets are on the left-hand side (LHS) of an assignment, they are for destructuring.
-
If brackets are inside the parenthesis used to define a function they are for destructuring.
Example of destructuring:
// 1) Destructure array items const [first, second,, fourth] = [10, 20, 30, 40]; // 2) Destructure object properties const { PI, E, SQRT2 } = Math;
These are both destructing because the brackets are on the LHS of the assignment.
Destructuring simply extracts named items out of an array (using their position) or properties out of an object (using their names) and into local variables in the enclosing scope. The 2 lines above are equivalent to:
// 1) assuming arr is [10, 20, 30, 40] const first = arr[0]; const second = arr[1]; // third element skipped const fourth = arr[3]; // 2) const PI = Math.PI; const E = Math.E; const SQRT2 = Math.SQRT2;
This is useful when you need to use a few properties out of a bigger object. For example, here’s a line to destructure Component
, Fragment
, and useState
out of the React’s API.
const {Component, Fragment, useState} = React;
After this line, you can use these React API objects directly:
const [state, setState] = useState();
When designing a function to receive objects and arrays as arguments, you can use destructuring as well to extract named items or properties out of them and into local variables in the function scope. Here’s an example:
const circle = { label: 'circleX', radius: 2, }; const circleArea = ({radius}, [precision = 2]) => (Math.PI * radius * radius).toFixed(precision); console.log( circleArea(circle, [5]) // 12.56637 );
I don’t really use arrays in function calls like the example above and neither should you. This example is only to demonstrate how you can destructure both objects and arrays in function arguments. Objects are more readable than arrays (when calling functions with them as inline arguments). |
The circleArea
function is defined to receive an object first argument and an array second argument. These arguments are not named and not used directly in the function’s scope. Instead, their properties and items are destructured and used in the function scope. You can even give destructured element default values (as it’s done for the precision
item).
In JavaScript, using destructuring with a single object as the argument of a function is an alternative to named arguments (which is available in other languages). It is much better than relying on positional arguments.