Template Strings
You can define strings in JavaScript using either single quotes or double quotes:
const greeting = "Hello World"; const answer = 'Forty Two';
These 2 ways to define string literals in JavaScript are equivalent. Modern JavaScript has a third way to define strings and that’s using the backtick character.
const html = ` <div> ${Math.random()} </div> `;
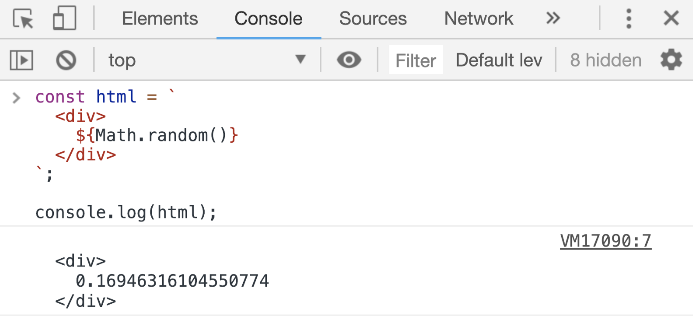
Strings defined with the backtick character are called template strings because they can be used as a template with dynamic values. They support expressions interpolation. You can inject any JavaScript expression within the ${}
syntax.
With template strings you can also have multiple lines in the string, something that was not possible with the regular-quoted strings. You can also “tag” templates strings with a function and have JavaScript execute that function before returning the string which is a handy way to attach logic to them. This tagging feature is used in the popular styled-components library (for React).
Backticks look very similar to single quotes, so make sure to train your eyes to spot template strings when they are used, and know when they’re needed.