The REST/SPREAD Syntax
Destructuring gets more interesting (and useful) when combined with the REST syntax and the SPREAD syntax, which are both done using the 3 dots (...
) syntax but they do different things.
If you’ve worked with React components, you might have used these 3 same dots to spread “props”. The JavaScript spread syntax was inspired by React (and others), but the meaning of the 3 dots in React/JSX and in JavaScript is different. |
The Rest syntax is what you use with destructuring. The spread syntax is what you use in object/array literals.
Here’s an example:
const [first, ...restOfItems] = [10, 20, 30, 40];
The 3-dots here, because they are in a destructuring call, represent a REST syntax. We are asking JavaScript here to destructure only 1 item out of this array (the first one) and then create a new array under the name restOfItems
to hold the rest of the items (after removing the first one).
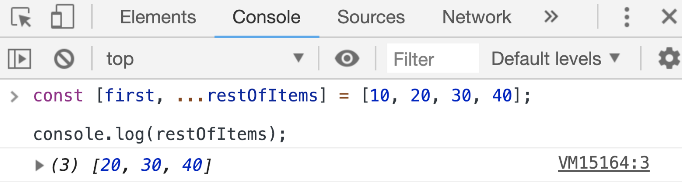
This is powerful for splitting the array and it’s even more powerful when working with objects to filter out certain properties from an object. For example, given this object:
const obj1 = { temp1: '001', temp2: '002', firstName: 'John', lastName: 'Doe', // 98 other properties };
If you need to create a new object that has all the properties of obj1 except for temp1
and temp2
, what would you do?
You can simply destructure temp1
and temp2
(and ignore them) and then use the rest operator to capture the remaining properties into a new object:
const { temp1, temp2, ...obj2 } = obj1;
How freaking cool is that?
REST syntax has nothing to do with REST APIs. Incidentally, when using REST APIs you end up with a lot of object properties that you do not need so you can use the REST syntax to pick the ones that you do. Have you looked at GRAPHQL yet?? |
The SPREAD syntax uses the same 3-dots to shallow-copy an array or an object into a new array or an object. This has nothing to do with destructuring but it’s commonly used to merge partial data structures into existing ones. It replaces the need to use the Object.assign
method.
const array2 = [newItem0, ...array1, newItem1, newItem2]; const object2 = { ...object2, newP1: 1, newP2: 2, };
When used in objects, property-name conflicts will resolve to taking the value of the last property. |